Apex
What is Apex?
Ans: Apex is a Strongly typed, object - oriented programming language. Apex works like a database scripts and stored procedures. The syntax of Apex looks like Java. Java is case sensitive but Apex is not case sensitive
- A Stored procedure is used to retrieve data, modify data, and delete data in database table. We don't need to write a whole SQL command each time you want to insert, update or delete in an SQL Database. A stored procedure is a precompiled set of one or more SQL statements which perform specific task.
- Apex enables us to write custom business logic to most system events, including button clicks, related record updates, and visualforce pages.
- Data types in Apex: Integer, Decimal, Double, Long, Date, DateTime, Boolean, String, Blob, sObject, Object.
- Apex provides built in support for
- DML calls to insert, update, delete a record.
- Inline SOQL or SOSL statements for retrieving records.
- Looping control structures that help with bulk processing.
- Object-Oriented Programming: It is a methodology or paradigm to design a program using Classes and Objects.
- Object: An Object can be defined as an instance of a class
- Object means a real time entity which has some characteristics, based on that characters we say what type of object it is.
- Example: Our PC. It has Keyboard, Screen, Touchpad based on these characters only we can say it is PC.
- Class: Collection of Objects, Methods, Variables, Constructors and Blocks is called class.
How to create Apex class?Ans: Syntax: public class className{
variables; constructors; Methods; } How to create an Object for class className objectNmae = new className();
What is a Variable?Ans: variables are used to store information A variable is a name given to a memory location. It is the basic unit of storage in a program. The value stored in a variable can be changed during program execution. Variable Declaration Syntax: AccessModifier Datatype VariableName = defaultValue(optional);- Local Variable : A variable defined with in a block or method or constructor is called local variable.
- Instance Variable: The variables declared inside the class are called Instance variables. This varaiables can be accessed anywhere in the class.
- If we want access Instance variables outside the class we have to use Object notation. ObjectName.VariableName
- Static Variable : A variable declared with static keyword are called Static variables.
- Static variables or methods can be accessed using ClassName only ClassName.Variable or MethodName.
Declaring a variable : Before we can use a variable in Apex we need to declare variable.Declaring variable means defining its type and optionally, setting an initial value.Every variable in Apex must have a name and its a data type.Data types means what type of the data, the variable will store.String Name = 'Venkanna'; What is Access Modifier?Ans: Access Modifier specifies the accessibility or scope of a Variable, Field, Method, Constructor or Class. Types of Access Modifiers- Private
- Public
- Protected
- Global
- Web Service
Access modifiers define the access level within the class. We have three types of access modifiers in Apex. These are public, private, and global.
Private:
If the access modifier is declared as ‘Private’, then this class will be local and this class cannot be accessed outside of that particular entity. By default, all the classes will have this modifier.
Public: If the access modifier is declared as ‘Public’ then this signifies that this class is accessible to the organization, along with the defined namespace.
Global: If the access modifier is declared as ‘global’ then this class will be accessible by all apex codes irrespective of the organization. What is Method?
Ans: A Method is a group of statements which are written in a sequential order to perform a specific task.
- A Method can return some value or return nothing
- A Method can be declared inside the class only.
- Syntax : AccessModifier ReturnType MethodName()
- Eg: public Integer add()
Types of Methods- Instance Method: Methods which are declared Inside class are called Instance Methods. These methods can be accessed through object only. Private methods can be accessed inside the class without any object.
- Static Method: Methods which are declared with Static keyword are called Static Methods.
- Virtual Method: The methods which are declared with Virtual keyword are called virtual methods.
public virtual class virtualParentClass{
public void add(Integer a, Integer b){ } public void sub(Integer c, Integer d){ } } * If we want override a virtual class we must use keyword extends. public class virtualChildClass extends virtualParentClass { } * The child class doesn't have any methods but, when we use extends all the methods which are declared in Parent class indirectly comes into exending class(ChildClass).* If the method is declared as private, it will not come into ChildClass.* If we want to override any method, that method should be declared with keyword Virtual public virtual Integer add(Integer a, Integer b) If we do not mention virtual keyword that method cannot be overridden.* In Child Class we must use override keyword if we want to override. public override Integer add(Integer a, Integer b)* In virtual methods overriding is optional(If we want we can override otherwise we cannot.)
4. Abstract Method: If we want to declare a Abstract class we must use keyword Abstract.
* Abstract methods should not have any body, but we can declare our methods.
* In virtual class overriding is optional, but in Abstract class if we declare any method we must and should override that method. * Let say we have declared 3 methods and overriding only 2 methods then it throws error. All the 3 methods must override.*Method overloading: If one method is implemented more than once with same name and different arguments and different return types then it is overloading.
What is Constructor?Ans: Constructor is like method which is used to perform task while creating an object. It is used to create an Object.- Constructor name should be same as ClassName.
- Constructor should not return any datatype even void also.
- If constructor declared as Private, Object cannot be created outside the class.
- Every class has it's own default constructor.
- Syntax : AccessModifier className(parameter)
- THIS keyword: It is used inside the constructor to access the same class instance variables and also used to call one method from other.
What are Collections in Salesforce?Ans: Collection is a group of elements which stores data into a single variable. We have three types of collections.- List
- Set
- Map
What is Difference between Normal FOR(integer i=0;i<=10;i++) loop and Enhanced FOR(Datatype variablename : collection) loop?
Ans : Normal for loop can iterates no of times that we specify in Condition. Ex: Suppose we have 1000 values to iterate but if we specify only 100 in Condition(i.e i<=100) then loop will iterate for 100 values only, remaining it will not iterate. If we Write Enhanced for loop, it will take the size of collections and iterates according to the size. Ex: If we have 10 values, it will iterate 10 times. If we have 200 values, it will iterate 200 times.
What is Apex?
Ans: Apex is a Strongly typed, object - oriented programming language. Apex works like a database scripts and stored procedures. The syntax of Apex looks like Java. Java is case sensitive but Apex is not case sensitive
- A Stored procedure is used to retrieve data, modify data, and delete data in database table. We don't need to write a whole SQL command each time you want to insert, update or delete in an SQL Database. A stored procedure is a precompiled set of one or more SQL statements which perform specific task.
- Apex enables us to write custom business logic to most system events, including button clicks, related record updates, and visualforce pages.
- Data types in Apex: Integer, Decimal, Double, Long, Date, DateTime, Boolean, String, Blob, sObject, Object.
- Apex provides built in support for
- DML calls to insert, update, delete a record.
- Inline SOQL or SOSL statements for retrieving records.
- Looping control structures that help with bulk processing.
- Object-Oriented Programming: It is a methodology or paradigm to design a program using Classes and Objects.
- Object: An Object can be defined as an instance of a class
- Object means a real time entity which has some characteristics, based on that characters we say what type of object it is.
- Example: Our PC. It has Keyboard, Screen, Touchpad based on these characters only we can say it is PC.
- Class: Collection of Objects, Methods, Variables, Constructors and Blocks is called class.
- Local Variable : A variable defined with in a block or method or constructor is called local variable.
- Instance Variable: The variables declared inside the class are called Instance variables. This varaiables can be accessed anywhere in the class.
- If we want access Instance variables outside the class we have to use Object notation. ObjectName.VariableName
- Static Variable : A variable declared with static keyword are called Static variables.
- Static variables or methods can be accessed using ClassName only ClassName.Variable or MethodName.
- Private
- Public
- Protected
- Global
- Web Service
Access modifiers define the access level within the class. We have three types of access modifiers in Apex. These are public, private, and global.
Private:
If the access modifier is declared as ‘Private’, then this class will be local and this class cannot be accessed outside of that particular entity. By default, all the classes will have this modifier.
Public: If the access modifier is declared as ‘Public’ then this signifies that this class is accessible to the organization, along with the defined namespace.
Global: If the access modifier is declared as ‘global’ then this class will be accessible by all apex codes irrespective of the organization.
What is Method?
Ans: A Method is a group of statements which are written in a sequential order to perform a specific task.
- A Method can return some value or return nothing
- A Method can be declared inside the class only.
- Syntax : AccessModifier ReturnType MethodName()
- Eg: public Integer add()
- Instance Method: Methods which are declared Inside class are called Instance Methods. These methods can be accessed through object only. Private methods can be accessed inside the class without any object.
- Static Method: Methods which are declared with Static keyword are called Static Methods.
- Virtual Method: The methods which are declared with Virtual keyword are called virtual methods.
- Constructor name should be same as ClassName.
- Constructor should not return any datatype even void also.
- If constructor declared as Private, Object cannot be created outside the class.
- Every class has it's own default constructor.
- Syntax : AccessModifier className(parameter)
- THIS keyword: It is used inside the constructor to access the same class instance variables and also used to call one method from other.
- List
- Set
- Map
Object Oriented Programming (OOP)
OOP(Object Oriented Programming) is a methodology that provides a way of modularizing a program by creating partitioned memory area for both data and methods that can be used as a template for creating copies of such modules (objects) on demand.
1.Encapsulation
2.Inheritance
3.Polymorphism
A good example of Inheritance in nature is parents producing the children and children inheriting the qualities of parents.
Data type :
2. Collections
3. Enums
1. Primitive data type :
These are the data types which are predefined by the Apex.- A primitive data types such as an Integer, Double, Long, Date, Date Time, String, ID or Boolean
- All primitive data types are passed by value, not by reference.
- All apex variable, whether they are class member variable, are initialised to null. Make sure that we initialise variable to an appropriate value before using them.
Apex primitive datatype include:-
- A value that can only be assigned true, false or null.
- A value that indicates a particular day date values contain no information about time. Date value must always be created with a system static method.
- Ex: Date myDate = Date.newinstance (2013,05,15);
- Output is 2012-05-15 00:00:00
- Ex: Time t1 = newInstance(19,20,1,20);
- Output is 19:20:01
- Date my = Datetime.now();
- Date t = Date.today();
The Date and Time classes also have instance methods for converting from one format to another :
- Ex: Time t2 = Datetime.now().time();
We can also manipulate the values by using a range of instance methods :
- Ex: Date t3 = Date.today(0);
- Date Next = t3.addDays(30);
2013-06-16 00:00:00
- To store numeric values in a variable, declare variables variable with one of the numeric data types. Integer, Long, Double and Decimal.
- A 32-bit number that doesn’t include a decimal point Integer have a minimum value of -2, 147, 467, 678 and a maximum value of 2,147, 483, 647.
Types of Operators:
Operators | Description | Syntax |
= | = operator (Assignment Operator) assigns the value of “y” to the value of “x”. | x = y |
+= | += operator (Addition assignment operator) adds the value of “y” to the value of “x” and then it reassigns the new value to “x”. | x += y |
*= | *= operator (Multiplication assignment operator)multiplies the value of “y” with the value of “x” and then it reassigns the new value to “x”. | x *= y |
-= | -= operator (Subtraction assignment operator) substract the value of “y” with the value of “x”, and then it reassigns the new value of “x”. | x -= y |
/= | /= operator (Division assignment operator) divides the value of “x” with the value of “y” and then reassigns the new value of “x”. | x /= y |
|= | |= operator(OR Assignment operator), If “x” (Boolean), and “y” (Boolean) both are false, then “x” remains false. | x |= y |
&= | &= operator, if “x” (Boolean), and “y” (Boolean) both are true, then “x” remains true. | x &= y |
&& | && operator (AND logical operator) it shows “short-circuiting” behavior that means “y” is evaluated only if “x” is true. | x && y |
|| | || OR logical operator. | x || y |
== | == Operator, if the value of “x” equals to the value of “y”, then the expression evaluates true. | x == y |
=== | === operator, if “x” and “y” reference the same location in the memory, then the expression evaluates true. | x === y |
++ | ++ Increment operator. | X ++ |
-- | -- Decrement operator. | x -- |
Comparison operators
Comparison operators, as the name suggests, deal with the process of comparing two values and return a Boolean value that indicates whether the comparison was true or false. The most commonly used comparison operators are as follows.
Equality ==
The double equals symbol compares whether two values are equal, for example:
In the above code the values on the left and right of the == are compared and if they are equal true is returned, otherwise false.
It should be noted that the comparison is by value and not by reference. So we are not checking that it is the same instance of a variable in memory, only that the variables have the same value, unless dealing with a user-defined type. This is a minor point for you to consider and for most developers will not need to be a concern, however, it is something you should be aware of.
Inequality !=
The inequality operator is the reverse of the equality operator above and compares two values to make sure that they differ:
Greater Than >
This operator compares two values and returns true if the left-hand value is larger than the right hand one:
Greater Than or Equal To >=
Similarly to the previous greater than the operator, this will return true if the left-hand value is greater than or equal to the right-hand value:
Less Than <
This is the reverse of the grater than the operator and returns true if the left-hand side is smaller than the right-hand side:
Less Than or Equal To
Again, similar to the previous less than operator but returns true if the left-hand side is less than or equal to the right hand side value:
Logical operators
Logical operators allow us to apply Boolean logic to combine multiple Boolean inputs to obtain a single Boolean output. There are three logical operators in Apex, AND, OR and NOT.
AND operator &&
The double ampersand && AND logical operator will return true if both the left-hand side and right-hand side are true. The operator will short-circuit so that if the left-hand value is false, the right-hand value will not be evaluated:
OR operator
The double pipe1 || OR logical operator will return a true value if either the left or the right-hand side are true. For the operator to return false, both the left and right-hand values must be false:
Not operator ! (logical complement)
The “not” (sometimes called logical complement) operator, returns the inverse value for a Boolean variable:
Assignment operators
Assignment operators allow us to assign and set values of variables. We have already seen the basic = operator that sets a variable to the value following the equals sign. We have some additional operators we can use to manipulate values as we assign them.
Addition assignment +=
The addition assignment operator will take the value on the right-hand side of the operator, add it to the left-hand side before assigning the new total to the variable on the left-hand side. For example:
If the variable is of type String, we can append another String onto the end using the operator as shown below:
Subtraction assignment -=
The subtraction assignment operator is similar to the addition operator in action but subtracts instead of adds the value on the right-hand side:
Multiplicative assignment *=
Multiplicative assignment works in the same way as both the additive and subtractive assignment but multiplies the left and right-hand sides before assigning the total as the new value:
Divisive assignment /=
The final assignment operator we will look at is the divisive assignment operator which divides the left-hand side by the right and then assigns the new value:
Example 1 :
{
Integer no; //======These are data members of class
String name; //=====
{
system.debug(‘roll no’ + no);
system.debug(’name’ + name);
}
}
Example 2 :
{
Integer exp; //===== variable /data members of the class
String department; //=====
{
//Write the logic here
}
}
- You must use one of the access modifiers for top-level class (Public or Global).
- You do not have to use access modifiers in the declaration of inner classes.
// The body of a class
}
Access Modifiers
Private:
- If you declare a class as a private, it is only known to the block in which it is declared.
- By default all the inner classes are private.
Public:
- If you declare a class as a public, this apex class is visible throughout your application and you can access the application anywhere.
Global:
- If you declare a class as a global, this apex class is visible to all the apex application in the application or outside the application.
- Note: If a method or a class (inner) is declared as global then the top level class also must be declared as global.
With Sharing:
- If you declare a class as a With Sharing, sharing rules given to the current user will be taken into consideration and the user can access and perform the operations based on the permissions given to him on objects and fields. (field level security, sharing rules)
Without Sharing:
- If you declare a class as a Without Sharing then this apex class runs in system mode which means apex code has access to all the objects and fields irrespective of current users sharing rules, fields level security, object permissions.
- Note: If the class is not declared as With Sharing or Without Sharing then the class is by default taken as Without Sharing.
- Both inner class and outer classes can be declared as With Sharing.
- If inner class is declared as With Sharing and top-level class is declared as without sharing, then the entire context will run in With Sharing context.
- Outer class is declared as With Sharing and inner class is declared as Without Sharing then inner class runs in Without Sharing context only. (Inner classes don't take the sharing properties from outer class).
Virtual:
- If a class is declared with keyword Virtual then this class can be extended (Inherited) or this class method can be overridden by using a class called overridden.
Abstract:
- This class contains Abstract methods, which will provide common method implementation to all subclasses.
Example 2:
Example 3:
=============================================
public without sharing class noSharing
{
//code
}
=============================================
Example 4:
=============================================
public with sharing class outer
{
//outer class code
without sharing class inner
{
//Inner class code
}
}
=============================================
In the above code outer class runs with current users sharing rules. But inner class runs with system context.
Example 5:
=============================================
public without sharing class outer
{
//Outer class code
with sharing class inner
{
//Inner class code
}
}
=============================================
In this way, both the inner and outer classes run with current users permissions.
- Modifiers such as public or final as well as static.
- The value of the variable.
- The name of a variable.
- The data type of the variable, such as String or Boolean.
Example :
- Modifiers, such as public or protected.
- The data type of the value returned by the method, such as String or Integer. Use void if the method does not return a value.
- A list of input parameters for the method, separated by commas, each preceded by its data type, and enclosed in parentheses ().If there are no parameters, use a set of empty parentheses.
- A method can only have 32 input parameters.
- The body of the method, enclosed in braces { }.
- All the code for the method, including any local variable declarations, is contained here.
Example 1 :
Example 2 :
- The object is an instance of the class. This has both state and behaviour.
- Memory for the data members is allowed only when you create an object.
- classname This is the name of class for which we are creating an object.
- objectname This is a reference variable.
- new This is a keyword which we are allocating the memory.
- () This is contructor.
In this way, you can use apex variable, method, object in salesforce.
Constructor
- Method name will be the same as a class.
- Access specifier will be public.
- This method will be invoked only once that is at the time of creating an object.
- This is used to instantiate the data members of the class.
1.Constructor are used to initialize the state of object,where as method is expose the behaviour of object.
2.Constructor must not have return type where as method must have return type.
3.Constructor name same as the class name where as method may or may not the same class name.
4.Constructor invoke implicitly where as method invoke explicitly.
5.Constructor compiler provide default constructor where as method compiler does't provide.
Example:-
public class Apple {
//instance variables
String type; // macintosh, green, red, ...
/**
* This is the default constructor that gets called when you use
* Apple a = new Apple(); which creates an Apple object named a.
*/
public Apple() {
// in here you initialize instance variables, and sometimes but rarely
// do other functionality (at least with basic objects)
this.type = "macintosh"; // the 'this' keyword refers to 'this' object. so this.type refers to Apple's 'type' instance variable.
}
/**
* this is another constructor with a parameter. You can have more than one
* constructor as long as they have different parameters. It creates an Apple
* object when called using Apple a = new Apple("someAppleType");
*/
public Apple(String t) {
// when the constructor is called (i.e new Apple() ) this code is executed
this.type = t;
}
/**
* methods in a class are functions. They are whatever functionality needed
* for the object
*/
public String someAppleRelatedMethod(){
return "hello, Apple class!";
}
public static void main(String[] args) {
// construct an apple
Apple a = new Apple("green");
// 'a' is now an Apple object and has all the methods and
// variables of the Apple class.
// To use a method from 'a':
String temp = a.someAppleRelatedMethod();
System.out.println(temp);
System.out.println("a's type is " + a.type);
}
}
For Example :
- 1. Default Constructor
- 2. Non-parameterized Constructor
- 3. Parameterized Constructor
1. Default Constructor
For Example:
=================================================
public class Example
{
}
Example e = new Example();
=================================================
In the above example, the apex class doesn't contain any constructor. So when we create an object for example class the Apex compiler creates a default constructor.
=================================================
public example()
{
}
=================================================
2. Non-parameterized Constructor
- It is a constructor that doesn't have any parameters is called Non-parameterized constructor.
- Parameters are nothing but the values we are passing inside a constructor.
3. Parameterized Constructor
- It is a constructor that has parameters.
- That means here we will take input from the user and then map it with our variable.
- Open developer console by clicking the org name on the Salesforce page.
- Click File --> New --> Apex Class.
- Enter the class name.
- Write the Apex Class.
integer Sal=10000;
Decimal bonus,salafterbonus;
for(bonus=5;bonus<=20;bonus+=5)
{
salafterbonus=sal+(sal*(bonus/100));
system.debug('salafterbonus'+bonus+'%bonus='+salafterbonus);
}
----------------------
for(integer i=20; i>=5;i-=5)
{
system.debug(i);
}
------------- ----------------------
public class Dog
{
public string Name='Scoopy';
public integer age=13;
public void disp()
{
system.debug('Name of the Dog'+Name);
system.debug('Age of my Dog'+age);
}
}
in Anonymous window :
Dog d1=new Dog();
system.debug(d1);
If else Statements
If else statements are used to control the conditional statement that are based on various conditions. It is used to execute the statement code block if the expression is true. Otherwise, it executes else statement code block.
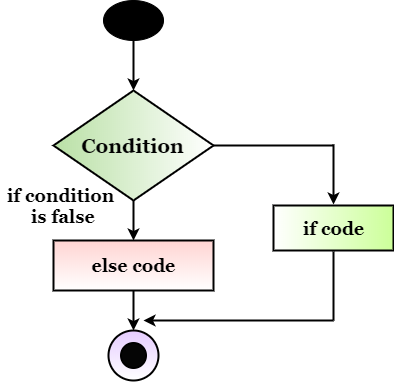
Syntax:if (Boolean_condition)
//Statement1
else
//statement 2
Example: Check the condition for voting
integer age=20;
if(age>=18) //checks the condition
{
System.debug('ELIGIBLE FOR VOTING');
}
else
{
System.debug('NOT ELIGIBLE');
}
------------- ------
Example:
integer marks=28;
if(marks>=33)
{
System.debug('PASS');
}
else
{
System.debug('FAIL');
}
--------------------------
Example: Comparing two different ages
integer age1=22; //my age
integer age2=20; //my brother`s age
if(age1>age2)
{
System.debug('I am elder');
}
else
{
System.debug('My brother is elder');
}
----------------------------
If else if Statement
Syntax:
if (expression1)
{
//statement
else if(expression2)
{
//statement
}
else if(expression3)
{
//statement
}
..
..
..
else
{
//statement
}
Example: Positive Number
integer num=10;
if(num>0)
{
System.debug('Number is positive');
}
else if(num<0)
{
System.debug('Number is negative');
}
else
{
System.debug('Number is Zero');
}
------------------------ --------------------------------
Example: Negative Number
integer num = (-50);
if(num>0)
{
System.debug('Number is positive');
}
else if(num<0)
{
System.debug('Number is negative');
}
else
{
System.debug('Number is Zero');
}
----------------------------- ---------------------
Example: Zero
integer num=(0);
if(num>0)
{
System.debug('Number is positive');
}
else if(num<0)
{
System.debug('Number is negative');
}
else
{
System.debug('Number is Zero');
}
----------------- ---------------------------------
Example:
integer place=1;
String medal_color;
if(place ==1)
{
medal_color='gold';
}
else if (place==2)
{
medal_color='silver';
}
else if(place==3)
{
medal_color='bronze';
}
else
{
medal_color='null';
}
System.debug('You have scored '+medal_color + 'Medal. Congratulations');
----------------- ---------------------------------
Example:
integer top_score=99,second_score=80,your_score=85;
if(your_score>top_score)
{
System.debug('You have scored the highest marks');
}
else if((your_score<top_score)&& (your_score>second_score))
{
System.debug('You have scored greater than the second score');
}
else if((your_score<top_score)&&(your_score<second_score)&&(your_score>65))
{
System.debug('You can do better');
}
else
{
System.debug('Work hard');
}
In the above example, we have created three integer variable top_score, second_score, your_score, and initialized them with 99, 80, 85 values.
In first if block, we are checking if your_score is greater than top_score i.e., 85>99, so the first if block will not get executed because the condition is false.
In the next else block, if your_score is less than top_score i.e., 99<85 (this condition is true) and the second condition is your_score is greater than second_score i.e., 85>80 (this condition is true).
There is a && operator. In the && operator, both the condition needs to be true. So, this block will get executed because both these conditions are true.
Once any of the blocks gets executed, the rest of the conditions will be skipped.
----------------- ---------------------------------
Example:
integer salary=250000;
Decimal bonus,salary_after_bonus;
if(salary>=200000)
{
bonus=15;
}
else if(salary>=150000)
{
bonus=12;
}
else if(salary>=10000)
{
bonus=10;
}
else
{
bonus=5;
}
salary_after_bonus=salary+(salary*(bonus/100));
System.debug('Salary '+salary);
System.debug('Bonus '+bonus+ '%');
System.debug('Salary after bonus'+ salary_after_bonus);

Example1:
integer i=1;
while(i<=10)
{
System.debug('Javatpoint');
i++;
}
In the above example, we have created a variable called (i) and initialized the value of the variable to 1, then, it checks while 1 is less than or equals to 10(condition is true). Now it will display “Javatpoint” on the screen. The increment operator (i++) will increase the value of (i) by 1.
Example2:
integer i=1;
while(i<=10)
{
System.debug('value of i= '+1);
i++;
}
------
Example3:
integer i=1;
while(i<=10)
{
System.debug('value of i= '+i);
i++;
}
---------------------
Example4:
integer i=0;
while(i<=100)
{
System.debug('value of i= '+i);
i+=10;
}
---------------------
Example5:
integer i=10;
while(i>=1)
{
System.debug('value of i= '+i);
i--;
}
For loop:
A for loop statement consumes the initialization, condition, and increment/decrement in one line by providing a shorter, easy to debug structure of looping.
Syntax:
for(initialization condition; testing condition; increment/decrement)
{
statement(s)
}

The initialization will only get executed once.
The condition and increment/decrement will be executed again.
Once it initialized, it will check for the condition, if the condition is true, then the statement will get executed, and again it will increment/decrement.
Once it increment/decrement, then again we check for the condition, if the condition is still true, it will get executed.
Once the condition becomes false, then the loop will stop further execution.
Example:
for(integer i=1;i<=5;i++)
{
System.debug('value of i= '+i);
}
Example:
integer salary=150000;
Decimal bonus,salary_after_bonus;
for(bonus=10;bonus<=25;bonus+=5)
{
salary_after_bonus=salary+(salary*(bonus/100));
System.debug('Salary after '+bonus+ '% bonus '+salary_after_bonus);
}
Example: Even no. in reverse order.
for(integer i=10;i>=0;i=i-2)
{
System.debug('value of i= '+i);
}
Three different types of for loop in Apex:
Traditional for loop
Syntax:
for (init_stml; ext_condition; increment_stmt)
{
code_block
}
Set iteration for loop
Syntax:
for (variable : list_or_set)
{
code_block
}
SOQL for loop
Syntax:
for (variable : [soql_query ])
{
code_block
}
Example:
List<String> stuNames=new List<String>
{'John', 'Sam', 'Barak'};
for(String stuName:stuNames)
{
System.debug('name= '+stuName);
}
Example:
List<Integer> empIds=new List<Integer>
{1200,96000,85274,74523,87523};
for(Integer empId:empIds)
{
System.debug('empId= '+empId);
}
Break and Continue Statement:
Break statement: The break statement is used to jump out of a loop.
Continue statement: The continue statement breaks one iteration in the loop (it will just skip the particular statement).
Example:
for(integer i=1;i<=20;i++)
{
if(i==10)
{
break;
}
System.debug('value of i= '+i);
}
In the above example, the break statement will come out of the loop once it hit the value 5.
Example:
for(integer i=1;i<=20;i++)
{
if(i==10)
{
continue;
}
System.debug('value of i= '+i);
}
In the above example, the continue statement skips the iteration (10) and jump to the next iteration.
Nested Loop:
Placing a loop inside another loop is called a nested loop.
Example:
for(integer i=0;i<=3;i++)
{
for(integer j=0;j<=2;j++)
{
System.debug('i ='+i+' j='+j);
}
}
Example:
for(integer i=1;i<=5;i++)
{
for(integer j=1;j<=i;j++)
{
System.debug(i);
}
}
------------------------ -------------------------
Static and Non Static Method
public class StaticExample
{
public static void method1()
{
system.debug('i m a static method');
}
public void method2()
{
system.debug('i m not a static method');
}
}
Static methods can be directly call with Class Name and dot operator
StaticExample.method1(); if run with this it runs fine and displays the result
------------------ ---------------------------------
Static and Non-static variable
---------------
Access Modifiers in Apex
----
public class Cat
{
private string name;
private integer size;
public void setname(string n)
{
name=n;
}
public void setsize(integer s)
{
if(s<=0)
{
system.debug('you cant set a wrong value for my cat');
size=10;
}
else
{
size = s;
}
}
public void display()
{
system.debug('Name of my Cat'+name);
system.debug('Size of my Cat'+size);
}
}
-------------------
Constructors in Apex
Yes, the constructor should always have the same name as the class.
Constructor looks like method but it is not. It does not have a return type and its name is same as the class name. Mostly it is used to instantiate the instance variables of a class./ used to set the values of the instance variables when you creating objects
public class Cat
{
private string name;
private integer size;
public Cat(string n, integer s) // Constructors with parameter
{
name=n;
size=s;
}
public Cat() // Constructors without parameter
{
name='Teena';
size=10;
}
public void display()
{
system.debug('Name of my Cat'+name);
system.debug('Size of my Cat'+size);
}
}
----------------------------
Inheritance in Apex
public virtual class vehicle {
public void model()
{
system.debug('these is the vehicle model');
}
public virtual void speed()
{
system.debug('this is speed for vehicle');
}
}
child class:
public class truck extends vehicle
{
public override void speed()
{
system.debug('these is the 50milesper/hr');
}
}
-------------------------------------------------
Collections in Apex (List, Set and Map)
Collections in Salesforce are various types that can contain numerous records. In other words, collections are groups of records . Collections have the ability to dynamically rise and shrink depending on the business needs. Collections in Apex can be lists, sets, or maps. There is no limit to the number of records that can be stored in a collection.
Salesforce has three types of collections:
- List collection
- Set collection
- Map collection
List Collection
A list can hold any type of data and is one of the most important types of collection. A list is an ordered collection of any data type such as primitive types, collections, sObjects, user-defined types, and built-in Apex types.
The following are the key features of a list collection in Salesforce:
- Duplicates and nulls are allowed in a list collection.
- “List” is the keyword to declare a list collection.
- In a list collection, the index position of the first entry is always zero (0).
- An Apex list collection has the ability to grow dynamically over time.
- In a list collection, the data type can be both primitive and non-primitive.
- The keyword followed by the data type has to be used within <> characters to define a list collection.
The syntax for a list is as follows:
List<datatype> listName = new List<datatype>();
List Class Salesforce: All methods required for a list collection are stored in the Apex list class.
What are the Methods in List?
In order to use lists in programming to achieve certain functions, a few methods in Salesforce are available.
The following are the list-class methods in Salesforce:
- Add: The values or items are added to the list using the list.add() method.
The syntax to use the “add” method is as follows:
List name.add();
Here is an example:
List<String> names =new List<String>();
names.add('Ram');
Here, we are adding the “Ram” value to the list “names” using the method “add”.
- Clone: It is the method of making a new record by using the details of an existent one. Simply put, it is creating a duplicate record.
The syntax to use the “clone” method is as follows:
NewList= Old list name. clone();
Here is an example:
New Names= names.clone();
In the above example, a new list “New Names” is being created, which is the clone of the list “names”.
- Remove: It is the method of removing the specific value of the list.
The syntax to use the “remove” method is as follows:
List Name.remove(index);
Here is an example:
List<String> names = new List<String>();
names.add('Ram');
names.add('John');
names.add('Sony');
names.remove(2);
By using “remove” syntax in the above-mentioned example, the second position value, which is “Sony”, is being removed.
- Size: It is the method of finding the number of elements present in the list.
The syntax to use the “size” method is as follows:
Listname. size();
You have to write the list name of which you want to find the size.
- Equals: This is the method of defining whether the value is equal or not. If the list and the specific list are equal, true is returned; otherwise, false is returned.
The syntax to use the “equals” method is as follows:
result=Listone.equals(Listtwo);
Here, “List two” is being compared with “Listone”. If both elements are the same, it shows true; if both elements are not the same, then it shows false.
- Get: This is the method that helps to return or find out a value from a list.
The syntax to use the “get” method is as follows:
String getlist= list.get(index);
In this syntax, you have to replace “list” with “list name” and “index” with “index number”.
- Set: This is the method that is used to change the element or value of an index.
The syntax to use the “set” method is as follows:
list.set(index, value);
The value of the index, which is mentioned in the code, will be changed to the value that is present in the code.
For example, if you write names.set(1, Mark);
Here the value at the “index 1” of the list of “names” will be changed to “mark”.
- Clear: This is the method that removes or deletes an element in the list.
The syntax to use the “clear” method is as follows:
list.clear();
These are a few popular methods. We have discussed Apex collections in Salesforce; now, we are going to discuss the next type of collection.
Set Collection
Set is an unordered collection. It is a unique set of values that do not have any duplicates. If you want no duplicates in your collection, then you should opt for this collection.
The following are the key features of a set collection in Salesforce:
- Any data types, such as primitive data types and sObjects, are allowed in a set collection.
- A set collection does not have an index.
- A set collection does not contain any duplicates or null values.
- Set collections are not widely used in Salesforce Apex.
- Sets can hold collections that are nested inside of one another.
- You have to use the set keyword followed by the primitive data type name within <> characters to declare a set collection.
The syntax for a set is as follows:
Set<datatype> setName = new Set<datatype>();
What are the Methods in Set?
The following are the set-class methods in Salesforce:
- Add(): Use the add method to add an element in a set collection.
The syntax for the “add()” method is as follows:
setName.add();
Here is an example:
Set <string> CitiesSet = new set <string>();
CitiesSet.add(Newyork); // Newyork will be added in the cities set.
- Remove(): This method is used to remove element(s) from a set collection.
The syntax for the “remove()” method is as follows:
SetName.remove();
Here is an example:
Set <string> CitiesSet = new set <string>();
CitiesSet.remove(Newyork); //Newyork will be removed from the cities set.
- Size(): Same as in list collections, the size method can be used to find the count of elements in a set.
The syntax for the “size()” method is as follows:
Setname. size();
- Contain(): This method is used to determine whether or not an element exists in a set or not.
The syntax for the “contain()” method is as follows:
Setname.contain(element);
- isEmpty(): This method is used to find if the element is zero or not.
The syntax for the “isEmpty()” method is as follows:
Setname.isEmpty();
These are some of the methods in a set collection. There are many other methods available in a set collection such as clear(), clone(), hashcode(), equals(), and many more.
Map Collection
Map is a key-value pair that contains each value’s unique key. It is used when something needs to be located quickly.
The following are the key features of a map collection in Salesforce:
- Any data type can be used for both the key and the value in a map collection.
- In a map collection, the null value can be stored in a map key.
- The keys of type string are case-sensitive in a map collection.
- You have to use the map keyword followed by the key and value data types enclosed in <> to declare a map collection.
The syntax for a map is as follows:
Map<datatype_key,datatype_value> mapName = new Map<datatype_key,datatype_value>();
What are the Methods in Map?
The following are the map-class methods in Salesforce:
- put(key, value): This method is used to add a value to a map collection.
The syntax for the “put(key, value)” method is as follows:
Map<integer, string> World Map = new Map <integer, string>();
World Map.put(1, ‘Australia’);
- get(key): This method helps to find the value of a key in a map collection.
The syntax for the “get(key)” is as follows:
Datatype_value = mapName.get(key);
- containskey(key): This method helps to declare that a map contains a specific key.
The syntax for the “containskey(key)” is as follows:
mapName.containskey(key);
- keySet(): This method is used to return a set containing all of a map’s keys.
The syntax for the “keySet()” is as follows:
Set<datatype_key> = mapName.keySet();
By now, you must have understood what exactly are collections in Salesforce with examples and how these collections can be used.
No comments:
Post a Comment